How Can You Effectively Determine Check and Uncheck States of Checkboxes in an Angular Tree?
In the world of web development, user experience is paramount, and one of the most intuitive ways to enhance interactivity is through the use of checkboxes. When it comes to managing hierarchical data structures, such as trees, the ability to check or uncheck items can significantly streamline user interactions. In Angular, a powerful framework for building dynamic web applications, implementing this functionality can seem daunting at first. However, with the right approach, you can create an engaging and user-friendly interface that allows users to effortlessly navigate and manipulate tree structures.
Understanding how to manage checkbox states in a tree structure is crucial for developers looking to build responsive applications. This involves not only tracking the individual states of checkboxes but also ensuring that parent and child relationships are respected. For instance, checking a parent node can automatically check all its child nodes, while unchecking it can do the opposite. This cascading behavior is essential for maintaining a clear and logical user interface, especially in applications that handle complex data.
As we delve deeper into the intricacies of managing checkbox states in Angular trees, we will explore various techniques and best practices. From leveraging Angular’s reactive forms to implementing custom logic for checkbox interactions, this guide will equip you with the knowledge needed to create a seamless and efficient user experience. Get ready to transform your
Understanding Checkbox States in Angular Tree Structure
Managing checkbox states in a tree structure in Angular involves understanding how to track the checked and unchecked states of each node effectively. This task can often require recursion or iterative methods to navigate the tree and update the states accordingly.
The first step is to define a data structure that represents your tree nodes. Each node should contain properties for the checkbox state, such as `isChecked`, `isIndeterminate`, and any necessary child nodes. A sample structure could look like this:
“`typescript
interface TreeNode {
id: number;
name: string;
isChecked: boolean;
isIndeterminate: boolean;
children: TreeNode[];
}
“`
Handling Checkbox Changes
When a checkbox state changes, it is essential to propagate this change throughout the tree. You can achieve this by creating functions that handle the checking and unchecking of nodes. The following methods can be useful:
– **Check Node**: When a node is checked, you can recursively check all its children.
– **Uncheck Node**: When a node is unchecked, you should recursively uncheck all its children.
– **Indeterminate State**: If a parent node has some but not all children checked, it should reflect an indeterminate state.
Here’s a basic implementation of these functions:
“`typescript
checkNode(node: TreeNode) {
node.isChecked = true;
node.isIndeterminate = ;
node.children.forEach(child => this.checkNode(child));
}
uncheckNode(node: TreeNode) {
node.isChecked = ;
node.isIndeterminate = ;
node.children.forEach(child => this.uncheckNode(child));
}
updateParentState(node: TreeNode) {
const checkedChildren = node.children.filter(child => child.isChecked);
const allChecked = checkedChildren.length === node.children.length;
node.isChecked = allChecked;
node.isIndeterminate = !allChecked && checkedChildren.length > 0;
}
“`
Example of Checkbox Handling in a Tree Component
To visualize how the checkboxes can be rendered and handled in an Angular component, consider the following example:
“`html
-
{{node.name}}
“`
In the component’s TypeScript file, manage the checkbox changes:
“`typescript
onCheckboxChange(node: TreeNode) {
if (node.isChecked) {
this.checkNode(node);
} else {
this.uncheckNode(node);
}
this.updateParentState(node); // Update parent states accordingly
}
“`
Table: Checkbox States Management
The following table outlines the states and actions for managing checkbox states in a tree structure:
Action | Node State | Child State |
---|---|---|
Check Node | Checked | All Checked |
Uncheck Node | Unchecked | All Unchecked |
Indeterminate State | Indeterminate | Some Checked |
By implementing these structures and functions, you can effectively manage checkbox states in a tree in Angular, ensuring a responsive and intuitive user interface.
Checkbox State Management in Angular Tree Structures
Managing the state of checkboxes in a tree structure within an Angular application involves several key steps. These include defining the tree structure, binding checkbox states to the model, and ensuring that interactions with parent checkboxes affect child checkboxes accordingly.
Defining the Tree Structure
The first step is to define the data structure for the tree. This typically involves a hierarchical representation using an array of objects. Each object can have properties for the node’s title, its checked state, and an array for child nodes.
“`typescript
interface TreeNode {
title: string;
checked: boolean;
children?: TreeNode[];
}
const treeData: TreeNode[] = [
{
title: ‘Parent 1’,
checked: ,
children: [
{ title: ‘Child 1.1’, checked: },
{ title: ‘Child 1.2’, checked: }
]
},
{
title: ‘Parent 2’,
checked: ,
children: [
{ title: ‘Child 2.1’, checked: },
{ title: ‘Child 2.2’, checked: }
]
}
];
“`
Template Binding
In the template, you can utilize Angular’s structural directives to display the tree. Using the `*ngFor` directive, bind the checkbox states to the `checked` property of each node.
“`html
“`
Checkbox Change Handler
Implement a method to handle checkbox changes. This method will update the state of child nodes based on the parent checkbox’s state.
“`typescript
onCheckboxChange(node: TreeNode): void {
if (node.children) {
node.children.forEach(child => {
child.checked = node.checked;
this.onCheckboxChange(child); // Recursively update child nodes
});
}
}
“`
Updating Parent Checkbox State
To ensure that a parent checkbox reflects the state of its children, you may need to implement logic that checks whether all child checkboxes are checked or unchecked. This can be done in the same change handler.
“`typescript
updateParentCheckbox(node: TreeNode): void {
if (node.children) {
const allChecked = node.children.every(child => child.checked);
const anyChecked = node.children.some(child => child.checked);
node.checked = allChecked; // Set parent checked state
}
}
“`
Integrate this method into your existing checkbox change handler to maintain consistency in the tree structure.
“`typescript
onCheckboxChange(node: TreeNode): void {
// Update child nodes
if (node.children) {
node.children.forEach(child => {
child.checked = node.checked;
this.onCheckboxChange(child);
});
}
// Update parent checkbox state
this.updateParentCheckbox(node);
}
“`
By following these structured steps, you can effectively manage the state of checkboxes in a tree structure within an Angular application. The combination of data binding, event handling, and recursive updates ensures that the UI remains in sync with the underlying data model.
Expert Insights on Managing Checkbox States in Angular Trees
Dr. Emily Tran (Senior Software Engineer, Angular Development Group). “To effectively manage checkbox states in a tree structure within Angular, it is crucial to implement a reactive approach using Angular’s reactive forms. This allows for dynamic updates and ensures that the check/uncheck states are accurately reflected in the model, facilitating better data handling.”
Mark Jensen (Frontend Architect, Tech Innovators Inc.). “Utilizing Angular’s Change Detection strategy can significantly enhance the performance when dealing with nested checkbox trees. By leveraging OnPush strategy and immutability, developers can optimize rendering and ensure that checkbox states are efficiently managed without unnecessary re-renders.”
Lisa Chen (UI/UX Specialist, Modern Web Solutions). “User experience is paramount when implementing checkboxes in a tree structure. It is essential to provide visual feedback for checked and unchecked states. Additionally, ensuring that the parent checkbox reflects the state of its children can enhance usability and clarity for users interacting with complex data hierarchies.”
Frequently Asked Questions (FAQs)
How can I implement checkboxes in a tree structure in Angular?
To implement checkboxes in a tree structure in Angular, you can use the Angular Material Tree component, which supports checkbox selection. You need to set up the tree data structure and bind the checkbox state to each node using Angular’s reactive forms or template-driven forms.
What is the best way to manage the state of checkboxes in a tree?
The best way to manage the state of checkboxes in a tree is by maintaining a model that reflects the checked status of each node. You can use a recursive function to traverse the tree and update the state based on user interactions, ensuring parent-child relationships are respected.
How do I handle parent-child checkbox selection in a tree?
To handle parent-child checkbox selection, you can implement logic that checks or unchecks all child nodes when a parent checkbox is toggled. Conversely, if all child nodes are checked, the parent checkbox should automatically be checked. This can be achieved by updating the state of each node accordingly.
Can I customize the appearance of checkboxes in the Angular tree?
Yes, you can customize the appearance of checkboxes in the Angular tree by using CSS styles or Angular Material themes. You can also create custom checkbox components to replace the default checkboxes, allowing for greater flexibility in design.
What libraries can assist with checkbox trees in Angular?
Several libraries can assist with checkbox trees in Angular, including Angular Material, ngx-treeview, and PrimeNG. These libraries provide pre-built components that simplify the implementation of tree structures with checkbox functionality.
Is it possible to disable checkboxes conditionally in an Angular tree?
Yes, it is possible to disable checkboxes conditionally in an Angular tree. You can bind the `disabled` property of the checkbox to a condition based on the node’s data or state, allowing for dynamic control over the checkbox availability.
Determining the check and uncheck states of checkboxes in a tree structure in Angular involves understanding the component architecture and state management. Angular provides various ways to handle checkbox states, particularly in hierarchical data representations like trees. Utilizing reactive forms or template-driven forms can facilitate the management of checkbox states effectively. It is essential to bind the checkbox states to a model that reflects the tree structure, ensuring that changes propagate correctly throughout the application.
One of the key insights is the importance of maintaining a consistent state across parent and child nodes. When a parent checkbox is checked or unchecked, it is crucial to update the states of its child checkboxes accordingly. This can be achieved through event binding and utilizing Angular’s change detection mechanisms. Additionally, implementing a service to manage the state can simplify the logic and enhance reusability across different components.
Another significant takeaway is the use of Angular’s built-in directives, such as *ngFor, to dynamically render the tree structure. This allows for a clean separation of concerns and promotes better maintainability of the code. Furthermore, employing strategies like lazy loading for large trees can improve performance, ensuring that the application remains responsive even with extensive datasets.
Author Profile
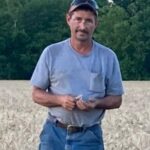
-
Hi, I’m Kendrik. This site is more than a blog to me. It’s a continuation of a promise.
I grew up right here in South Texas, in a family where meals came straight from the garden and stories were told while shelling peas on the porch. My earliest memories are of pulling weeds beside my grandfather, helping my mother jar pickles from cucumbers we grew ourselves, and learning, season by season, how to listen to the land.
Here at BrownsvilleFarmersMarket.com, I share what I’ve learned over the years not just how to grow crops, but how to nurture soil, nourish health, and rebuild food wisdom from the ground up. Whether you’re exploring composting, greenhouse farming, or hydroponic setups in your garage, I’m here to walk with you, row by row, one honest post at a time.
Latest entries
- April 26, 2025PlantsWhen Is the Best Time to Plant Cereal Rye for Deer?
- April 26, 2025PlantsDoes Wet And Forget Really Kill Plants? Unraveling the Truth Behind the Product!
- April 26, 2025HarvestingWhen Should You Harvest Your Yukon Gold Potatoes for Optimal Flavor?
- April 26, 2025Tree NurturingDo Palm Trees Have Big Roots? Exploring the Truth Behind Their Root Systems